A dimension that was working fine on SQL server analysis server started to give the following error :
OLE DB Error: OLE DB or ODBC error: Query timeout expired; HYT00.
After doing the following the dimension processes just fine now. There is a timeout for external data queries defined in the sql server analysis services properties. You can get to it from ssms - right click the analysis server as shown and select properties:
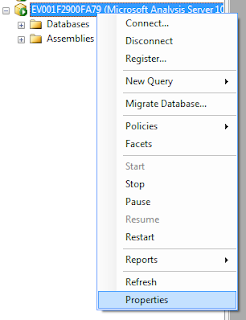
And then select "Show Advanced (All) Properties" as shown:
Edit the current value of "ExternalCommandTimeout" as shown:
And click Ok.
You can open the Properties / Show Advanced view again to verify that the settings took:
Enjoy!
OLE DB Error: OLE DB or ODBC error: Query timeout expired; HYT00.
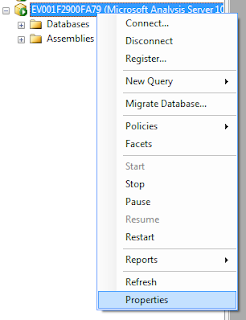
And then select "Show Advanced (All) Properties" as shown:
Edit the current value of "ExternalCommandTimeout" as shown:
And click Ok.
You can open the Properties / Show Advanced view again to verify that the settings took:
Enjoy!